Python uses variables to store data in memory. Think of variables as containers that hold data which can be changed later in the program.
For example:
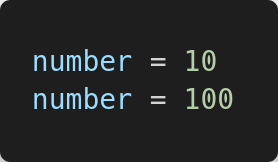
Initially the value of number was 10. Later it was changed to 100.

Python is a case-sensitive language and variable names should use snake case¹, like so:

Always give variables a name that makes sense.
While c = 10 is valid, writing count = 10 would make more sense. It is easier to figure out what the variable represents when you revisit your code and for other programmers on your team.
It’s time for a challenge! Go to the playground and replace the ??? such that 'Python' is printed.
Fundamental Data Types
Everything in Python has a data type, or class². The ones you need to know about now are:
Integers — any whole number. Integers belong to the int class:

Floats — any decimal number. Floats belong to the float class:

For all you mathematicians out there, there’s one more numerical data type to be aware of: complex³. For everyone else, you don’t need to worry about these 😅!
Strings — any character wrapped in single or double quotes ('' or ""). Strings belong to the str class:
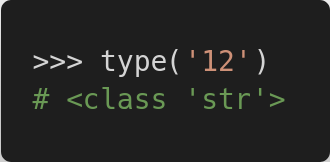
By using single quotes you can embed double quotes and vice-versa:

Though it’s more pythonic⁴ to use single quotes until you need double quotes.
We can use Python’s built-in type() function, passing an argument in parenthesis, to see the data type, or class, of our argument.
Footnotes
[1] Snake case is a writing convention where words are separated by underscores. It typically uses all lower case letters.
[2] A class is a code template where objects are created. For example, we may have a Car class which acts as a factory for making unique car objects. These cars will belong to the Car class.
[3] Complex numbers come in the form a + b*j, where a is a real number, b is an imaginary number and j represents √−1.
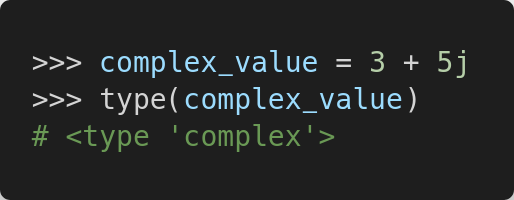
[4] Pythonic is an adjective used to describe code that is clear, concise and maintainable.
Pythonic means code that doesn’t just get the syntax right but that follows the conventions of the Python community and uses the language in the way it’s intended to be used.
Learn More
